
You can fix this by clicking on the red circle and then Fix. An empty implementation adversely affects performance during animation.Ĭut and paste all the lazy vars from the view controller to our new view.īelow the last lazy var, override init(frame:), by typing init and then choosing the first autocomplete result from Xcode.Īn error will come up saying `’required’ initializer ‘init(coder:)’ must be provided by subclass of ‘UIView’: Only override draw() if you perform custom drawing. Now click Next, then Create, and remove the commented code:
#Appdelete make tab bar vc root window swift 4 code
This is just to tell Xcode to automatically make our class inherit from UIView and it will add some boilerplate code (that we’ll probably remove anyway in the case of UIView). Now write “ProfileView” as the name of the class and next to “Subclass of:” make sure to type in “UIView”. Now right click on the ContactCard folder in the Project Navigator and choose New File:Ĭlick on Cocoa Touch Class and then click Next. More on MVC Model-View-Controller (MVC) in iOS: A Modern Approach. The reason why we’re doing this is to follow the Model-View-Controller architectural pattern, or MVC for short. We can do this by subclassing UIView and moving all of our UI elements there. Go ahead and do this before running the application and the result should look like this:īefore proceeding to creating UI elements programmatically, we’ll need to slim down our ViewController.swift file to be able to only accommodate actual logic instead of just code for UI elements. This will add the word “Profile” to the navigation bar so the user knows where they currently stand. You can also add this line self.title = "John Doe" in viewDidLoad. Self.navigationController?.navigationBar.isTranslucent = false Now, go to viewDidLoad method and add this line self.navigationController?.navigationBar.isTranslucent = false below super.viewDidLoad() so it will look like this: UINavigationBar – UIKit | Apple Developer Documentation UINavigationController – UIKit | Apple Developer Documentation I also recommend to check out, and read thoroughly, Apple’s docs on the UINavigationController and UINavigationBar:

I will personally go with the second choice, but please, go ahead and explore the first one. Doing this will make the navigation bar opaque (in case you didn’t notice, it is a little bit transparent) and now the superview’s top edge will be the bottom of the navigation bar. Set the navigation bar’s isTranslucent property to false.

A UINavigationController would easily solve this with its navigation bar.Ī UINavigationController is simply a stack on which you push many views as you go further into the application.
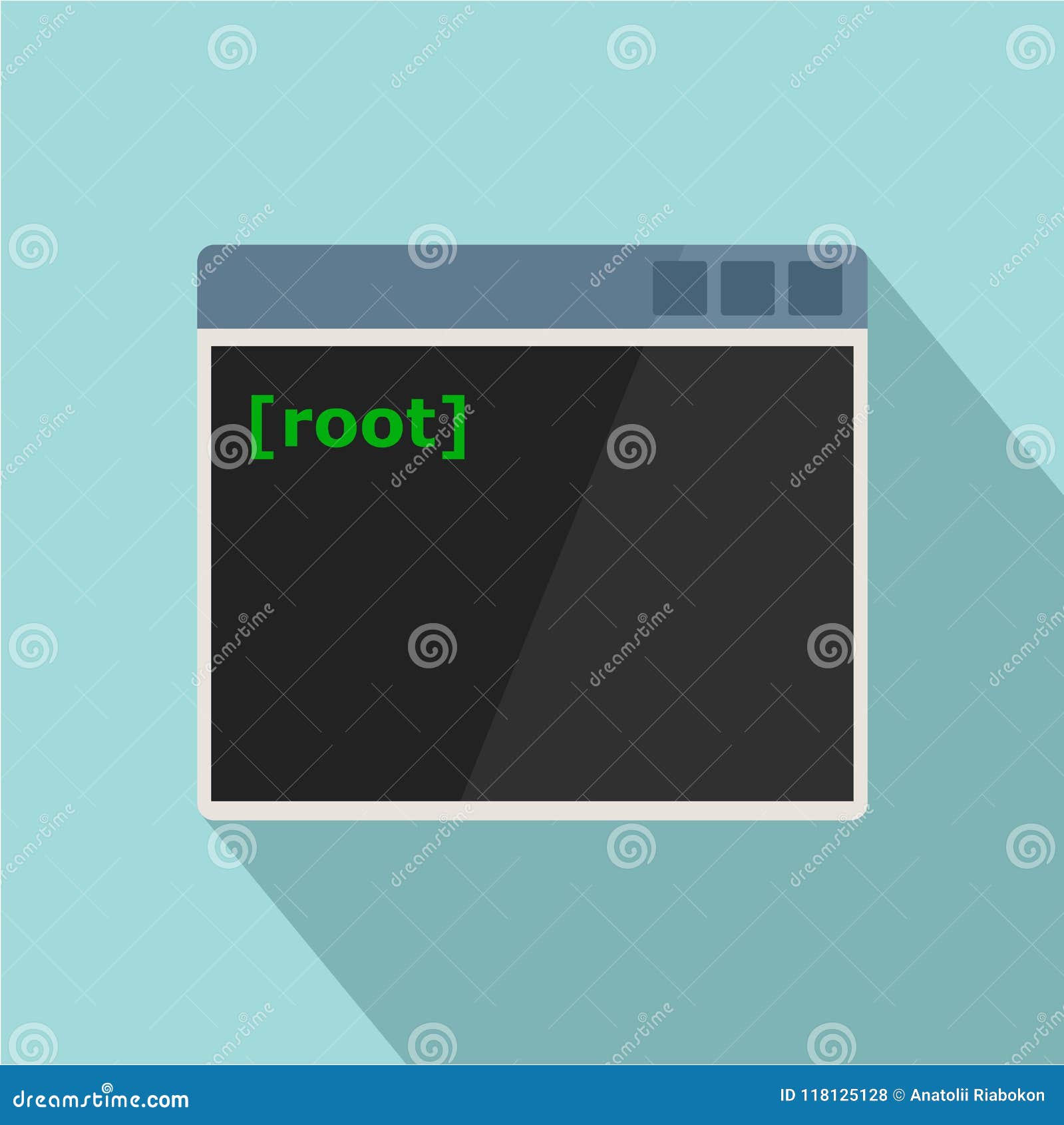
In an app like this, you’ll probably want a navigation bar so that a user can navigate from the list of contacts to a detail view for a certain contact and then go back to the list. In this tutorial, we will cover some of the most used UI elements in all applications: In the first part, we built a simple mobile application’s UI completely in code without the use of Storyboards or NIBs. Welcome to part two of Creating UI Elements Programmatically Using PureLayout.
